Some GitHub Actions Tips
I’m using GitHub Actions frequently enough to know where to look for things and which features are available, but infrequently enough to forget how things are done. Good thing, all my actions are available in GitHub, so I can look things up when I need to.
GitHub Actions are great for a CI/CD and automation platform, integrate great into GitHub itself and offer a generous free tier even for private repositories. I have some trouble with the documentation and just don’t find anything in there or have trouble understanding stuff. So I’ll collect some things here to find them again in the future.
Run GitHub Actions From the Web Interface
This was the most annoying thing for me, not being able to re-run a job — failed or not — through the web interface. I have no idea where I found this bit, but it stuck with me ever since. Just do this in your workflow .yaml definition:
name: build
on:
workflow_dispatch:
workflow_dispatch makes it possible to trigger a run on github.com directly.
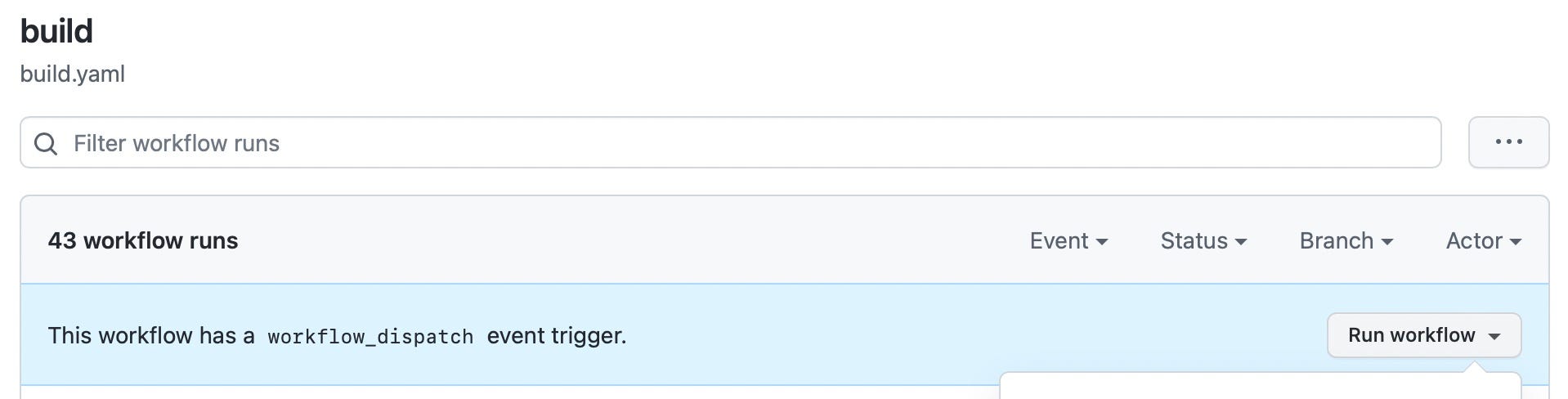
Use a Cron Pattern
I build a few containers which are updated from time to time and I add some extras to them, so I pull them down, do my modifications and push them back to the GitHub Registry, so I can use them in my k3s cluster. To keep them updated automatically, either on the :latest tag (you should know when to do that) or to receive all patch updates, something like :v5 I have a GitHub Action running every week to update my packages.
To run an action occasionally, based on cron patterns, just do this in your .yaml definition:
on:
schedule:
- cron: '23 */2 * * *'
This GitHub Action above will run every two hours at minute 23, so at 6:23, 8:23, 10:23 and so on. Crontab.guru is a great resource to build your own cron patterns.
Only Perform Changes When Files or Directories Changed
By default, GitHub Actions run on whatever is configured in the on part of the workflow, usually when a push or pull request is being made, no matter to which part of the repository. Sometimes I just update a lot of markdown files and no other code in a repository, so I don't need GitHub Actions to run when these are being updated. Me being me, I sometimes commit and push a repository 10 times in a row with just simple text changes. I certainly do not need 10 GitHub Actions workers running.
To ignore folders or files completely, you can just do this:
on:
push:
branches:
- main
paths-ignore:
- "**.md"
- "directory/**"
The paths-ignore part is important here, updates to these files and folders will never trigger an Action.
However, if you want some more advanced stuff, there’s an action you can pull in to check if files have been modified:
verify-changed-files:
Github action to detect file changes that occur during the workflow execution.
The documentation for this action is available on GitHub itself.
Here’s an example to check if files in a folder have been changed and either execute the full action or skip a part:
- name: Verify Changed files
uses: tj-actions/[email protected]
id: verify-changed-files
with:
files: |
dockerfiles/gollum
- name: Perform action when dockerfiles/gollum changes
if: contains(steps.verify-changed-files.outputs.changed_files, 'dockerfiles/gollum')
run: |
- name: build gollum image
uses: docker/build-push-action@v2
with:
context: dockerfiles/gollum
platforms: linux/amd64,linux/arm64,linux/arm/v7
push: true
This will check if anything changed within the dockerfiles/gollum directory and only executes the docker/build-push-action if changes were detected, otherwise it'll be skipped. This is a huge time saver to not build a Docker Image when no changes were made.